Manipulating the DOM with JavaScript Part 2 (Modern Tools and Techniques)
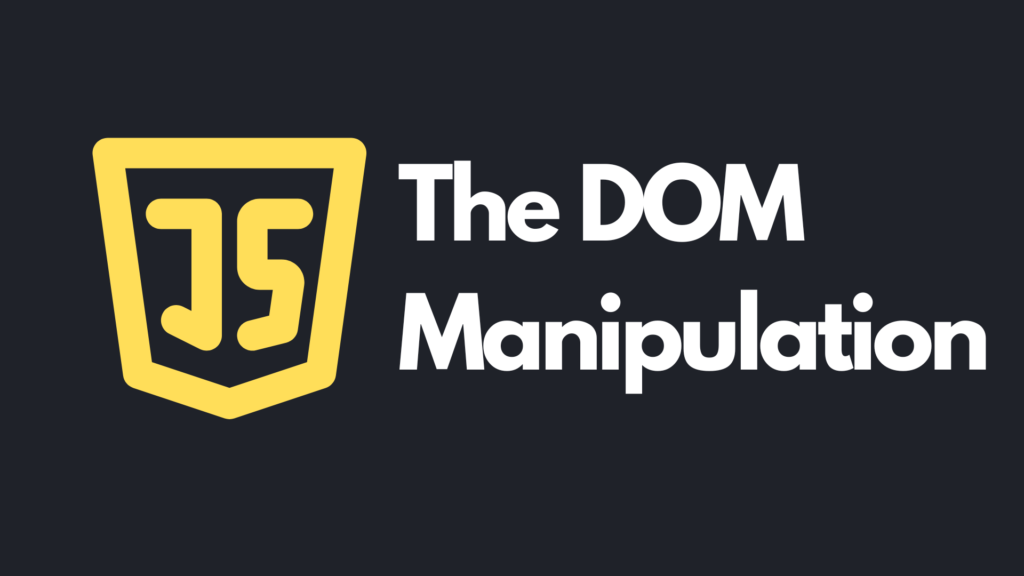
alitechguru.com
Introduction
This is the second series on the DOM in Javascript. In the first series, you learnt about the DOM, various DOM methods and events. If you haven’t gone through the first article, kindly click here to read it before you continue.
Since the history of web development, several DOM selector methods have been used to control the DOM. These methods are not suitable for all use cases. Do you know why? When engineers are building out very robust applications, using this vanilla approach is not clean and elegant. There is a need to write code that is clean, scalable and easy to maintain. This led to the modern ways of manipulating the DOM.
This article is primarily for beginners. However, intermediate and advance level developers will find it very useful.
In this article, you will go beyond wrestling with awkward selectors and learn modern approaches to manipulating the DOM. This will help you to write clean and scalable code while you manipulate the DOM.
Below is the outline of what this article covers:
- Beyond vanilla JavaScript.
- Virtual DOM (React’s secret weapon).
- JSX (React’s superpower).
- Vue templates.
The vanilla approach to manipulating the DOM leverages several methods like getElementById, document.querySelector and their other companions. While this approach remains trustworthy to date, cutting edge libraries and frameworks like React offer game-changing alternatives.
React Virtual DOM (React’s secret weapon)
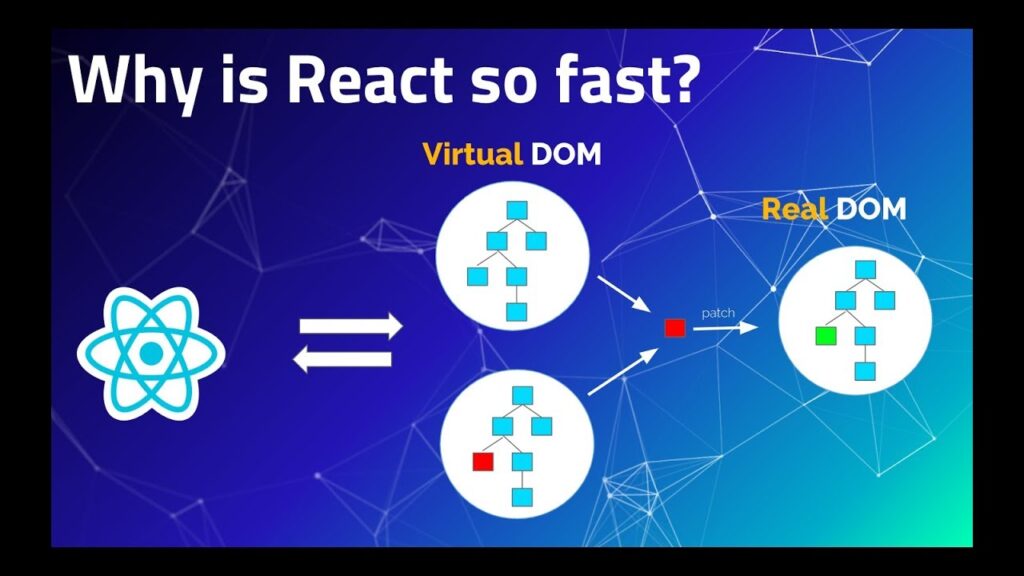
Introduction to React by Imran Sayed
Have you ever wondered why the fuzz and wide adoption of the React framework?
Have you imagine a lightweight shadow copy of the real DOM, where you describe your desired changes. React efficiently updates only the necessary parts, boosting performance and keeping your code clean?
This here (the virtual DOM) is one of the reasons React is very fast.
JSX (Reacts superpower)
Say goodbye to switching back and forth between HTML and Javascript. JSX lets you write HTML-like structures right within your JavaScript, blurring the lines between code and UI for a seamless development experience.
Let’s see some code snippets in action. This will help you to get a practical insight into how React and Vue handle DOM manipulation better than the traditional way vanilla JavaScript does.
Imagine you want to add a “Hello, world!” paragraph to your page. Here’s how it looks in Vanilla JS, React and Vue:
Vanilla javaScript
const newElement = document.createElement('p');
newElement.textContent = 'Hello, world!';
document.body.appendChild(newElement);
In the code snippet above, you created a paragraph and stored it in a constant variable called newElement. Line 2 added the text ‘Hello, world!’ to the paragraph, and lastly, line 3 added the paragraph to the body of the page.
Now let’s see how you would do this same thing in Reactjs:
React.js
const AddParagraph = () => {
return (
<div>
<p>Hello, world from React!</p>
</div>
);
}
In the React code snippet above, you have a typical es6 function, that returns a jsx. The “Hello, world from React!” paragraph is inside the Jsx. You can see that there was no need for those inelegant-looking methods like document.createElement. This approach is very clean and readable. You can go further to add more UI elements, add an event listener, or add a function that triggers when the event is initiated. Then do something once the function is called.
Moreover, you can also write your logic just above the Jsx to control the DOM.
Vue templates.
Do you prefer a clear separation of concerns? Vue’s got you covered. Embed Javascript expressions within your HTML templates to define dynamic behavior without cluttering your logic.
This is how you would write the same paragraph in vue.
Vuejs
<template>
<div id="app">
<p @click="handleParagraphClick">Hello, world from Vue!</p>
</div>
</template>
<script>
export default {
setup() {
const handleParagraphClick = (event) => {
console.log('Paragraph clicked!', event);
// Your custom logic here
};
return { handleParagraphClick };
}
};
</script>
This code defines a simple Vue component with a single paragraph element containing the text “Hello, world from Vue!”. Here’s a breakdown:
- <template>: This tag wraps the HTML template for the component.
- <p>: This tag defines a paragraph element.
- “Hello, world from vue!”: This is the text content displayed within the paragraph element.
- @click : Th
is is a
directive added to the<p>
element to bind thehandleParagraphClick
method to the click event. Clicking the paragraph calls the handleParagraphClick, method. With this, you can easily manipulate the DOM.
Conclusion
In this article, you have learnt about the modern approaches used to manipulate the DOM. You have discovered the powerful secret behind React speed and performance, the virtual DOM. You are now acquainted with some of the foundations to write clean and scalable code while you manipulate the DOM.
Moreover, you have seen that while the traditional way of DOM manipulation is still very trustworthy, and has its use case. The modern approach of manipulating the DOM as shown in React and Vue frameworks is the best option for very large and robust applications.
In our next article, you shall explore more advanced ways of manipulating the DOM using the modern approach. Stay tuned. Feel free to share this article far and wide.
Links to resources for further study
Check out some resources below to learn more:
Thanks for reading this article. Also, kindly follow me on Twitter (X) here, and let’s connect on Linkedin here. See you in my next article.
Recent Comments